- composer create-project laravel/laravel:^9.* AppNameHere
Now create a databse and put credeintials into .env file like below
.env
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=databasename
DB_USERNAME=dadtabaseusername
DB_PASSWORD=databasepassword
Now we will install laravel default authentication system Breeze.
- composer require laravel/breeze --dev
- php artisan breeze:install
- php artisan migrate
- npm install
- npm run dev
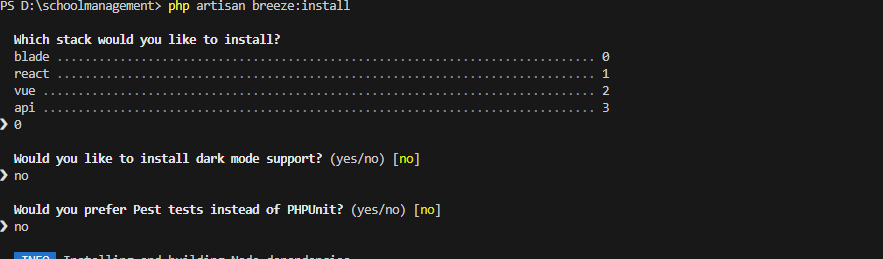
Now go to the database folder and find the users table and under the Schema paste the table structure like below
$table->id();
$table->string('name');
$table->string('username')->nullable();
$table->string('email')->unique();
$table->timestamp('email_verified_at')->nullable();
$table->string('password');
$table->string('photo')->nullable();
$table->string('phone')->nullable();
$table->text('address')->nullable();
$table->enum('role',['admin','vendor','user'])->default('user');
$table->enum('status',['active','inactive'])->default('active');
$table->rememberToken();
$table->timestamps();
Now run the command
php artisan migrate
npm install
Make sure before run the npm command you have to installed nodejs package on your system.
Now we seed Demo data, follow our step
php artisan make:seeder UsersTableSeeder
Now go to the seeder folder and find the newly created seeder UsersTableSeeder.php and paste the below code.
use Illuminate\Support\Facades\Hash;
use DB;
Use both Hash and DB Hash will be use for encrypt password and DB facades for call the DB table.
public function run()
{
DB::table('users')->insert([
//Admin
[
'name'=>'Admin',
'username'=>'admin',
'email'=>'admin@gmail.com',
'password'=>Hash::make('111'),
'role'=>'admin',
'status'=>'active',
],
//Vendor
[
'name'=>'Pallab Vendor',
'username'=>'vendor',
'email'=>'vendor@gmail.com',
'password'=>Hash::make('111'),
'role'=>'vendor',
'status'=>'active',
],
//User or Customer
[
'name'=>'Advik User',
'username'=>'advik',
'email'=>'user@gmail.com',
'password'=>Hash::make('111'),
'role'=>'user',
'status'=>'active',
],
]);
}
Now we will generate fake data, so go to the Models folder and find the User.php file and make it all fillable property.
protected $guarded = [];
Now go to the databse folder and under the database folder find factories folder and find the UserFactory.php file and create some fake data as per you table fields.
public function definition()
{
return [
'name' => fake()->name(),
'email' => fake()->unique()->safeEmail(),
'email_verified_at' => now(),
'password' => '$2y$10$92IXUNpkjO0rOQ5byMi.Ye4oKoEa3Ro9llC/.og/at2.uheWG/igi', // password
'phone'=>fake()->phoneNumber,
'address'=>fake()->address,
'photo'=>fake()->imageUrl('60','60'),
'role'=>fake()->randomElement(['admin','vendor','user']),
'status'=>fake()->randomElement(['active','inactive']),
'remember_token' => Str::random(10),
];
}
Now go the seeders folder and open the file DatabseSeeder.php file and call the UserTableSeeder like below
public function run()
{
$this->call(UsersTableSeeder::class);
\App\Models\User::factory(10)->create();
// \App\Models\User::factory()->create([
// 'name' => 'Test User',
// 'email' => 'test@example.com',
// ]);
}
Now run the below artisan command
php artisan migrate:fresh --seed